最近在学习go时,看到微服务rpc时,在想php能不能实现呢,答案是肯定的,下面写下来记录一下。
先看目录结构
rpc
----api
--------Test.php
----client
--------RpcClient.php
--------RpcJsonClientGo.php
----server
--------RpcServer.php
1、外面先实现php的rpc服务
RpcServer.php
<?php
class RpcServer
{
private $params = [
'host' => '',
'port' => '',
'path' => ''
];
private $config = [
'real_path' => '',
'max_size' => 2048
];
private $server = null;
public function __construct($params)
{
$this->check();
$this->init($params);
}
private function check()
{
$this->serverPath();
}
private function init($params)
{
$this->params = $params;
$this->createServer();
}
private function createServer()
{
$host = $this->params['host'] ?? '';
$port = $this->params['port'] ?? 8081;
if(empty($host)){
exit('host error');
}
$this->server = stream_socket_server("tcp://{$host}:{$port}", $errno, $errstr);
if (!$this->server) exit([
$errno, $errstr
]);
}
public function serverPath()
{
$path = $this->params['path'];
$realPath = realpath(__DIR__ . $path);
if ($realPath === false || !file_exists($realPath)) {
exit("{$path} error!");
}
$this->config['real_path'] = $realPath;
}
public static function instance($params)
{
return new RpcServer($params);
}
public function run()
{
echo "开始服务......\n";
while (true) {
$client = stream_socket_accept($this->server);
if ($client) {
echo "有新连接\n";
$buf = fread($client, $this->config['max_size']);
print_r('接收到的原始数据:' . $buf . "\n");
$this->parseProtocol($buf, $class, $method, $params);
$this->execMethod($client, $class, $method, $params);
fclose($client);
echo "关闭了连接\n";
}
}
}
private function execMethod($client, $class, $method, $params)
{
if ($class && $method) {
$class = ucfirst($class);
$file = $this->params['path'] . '/' . $class . '.php';
if (file_exists($file)) {
require_once $file;
$obj = new $class();
if (!$params) {
$data = $obj->$method();
} else {
$data = $obj->$method($params);
}
$this->packProtocol($data);
fwrite($client, $data);
}
} else {
fwrite($client, 'class or method error');
}
}
private function parseProtocol($buf, &$class, &$method, &$params)
{
$buf = json_decode($buf, true);
$class = $buf['class'];
$method = $buf['method'];
$params = $buf['params'];
}
private function packProtocol(&$data)
{
$data = json_encode($data, JSON_UNESCAPED_UNICODE);
}
}
RpcServer::instance([
'host' => '127.0.0.1',
'port' => 8081,
'path' => '../api'
])->run();
Test.php
<?php
class Test
{
public function testString()
{
return '测试字符串方法';
}
public function testParams($params)
{
return $params;
}
public function getName(){
return 'hello word ' ;
}
}
2、在写客户端
RpcClient.php
<?php
class RpcClient
{
private $urlInfo = array();
public function __construct($url)
{
$this->urlInfo = parse_url($url);
}
public static function instance($url)
{
return new RpcClient($url);
}
public function __call($name, $arguments)
{
$client = stream_socket_client("tcp://{$this->urlInfo['host']}:{$this->urlInfo['port']}", $errno, $errstr);
if (!$client) {
exit("{$errno} : {$errstr} \n");
}
$data = [
'class' => basename($this->urlInfo['path']),
'method' => $name,
'params' => $arguments
];
fwrite($client, json_encode($data));
$data = fread($client, 2048);
fclose($client);
return $data;
}
}
$client = new RpcClient('http://127.0.0.1:8081/test');
echo $client->testString() . "\n";
echo $client->testParams(array('name' => 'tuzisir', 'age' => 23));
查看运行结果
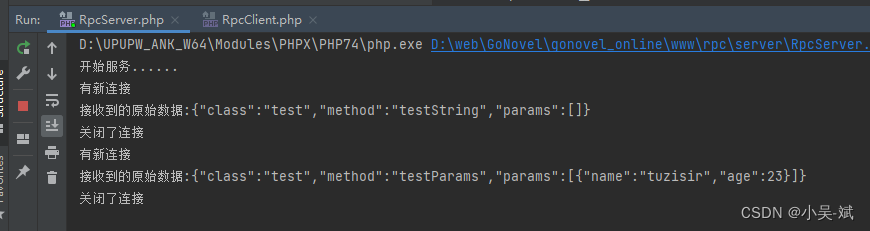
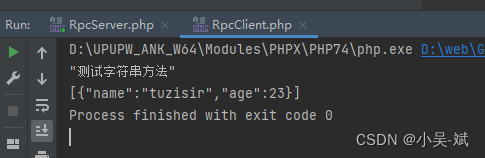
下面跨语言连接Go的jsonrpc服务
RpcJsonClientGo.php
<?php
class RpcJsonClientGo
{
private $conn;
function __construct($host, $port)
{
$this->conn = fsockopen($host, $port, $errno, $errStr, 3);
if (!$this->conn) {
return false;
}
}
public function Call($method, $params)
{
if (!is_scalar($method)) {
return "Method name has no scalar value";
}
if (!$this->conn) {
return false;
}
$err = fwrite($this->conn, json_encode([
'method' => $method,
'params' => array($params),
'id' => 0,
]) . "\n");
if ($err === false) {
return false;
}
stream_set_timeout($this->conn, 0, 3000);
$line = fgetc($this->conn);
if($line === false){
return null;
}
return json_decode($line,true);
}
}
$client = new RpcJsonClientGo("127.0.0.1",8080);
echo $client->Call(['ddd'=>22], "this is php languages");
执行php客户端文件,在go服务端就能看到发送来的数据
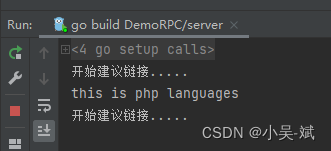